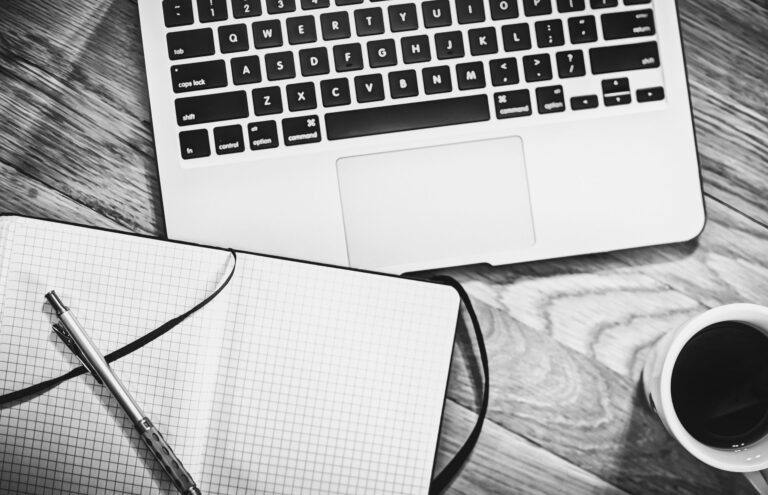
CSS Layout
Introduction When building a webpage, understanding layouts is key to creating a visually appealing and…
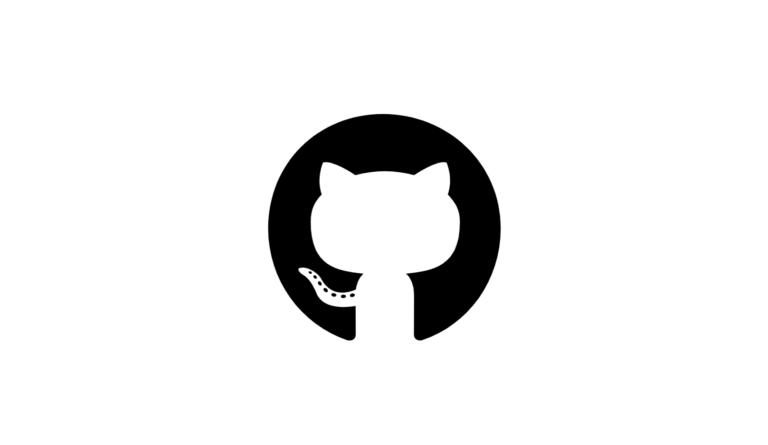
Deploy a Static Site on Github
The following tutorial shows how to deploy a static site using Github Pages. This assumes…
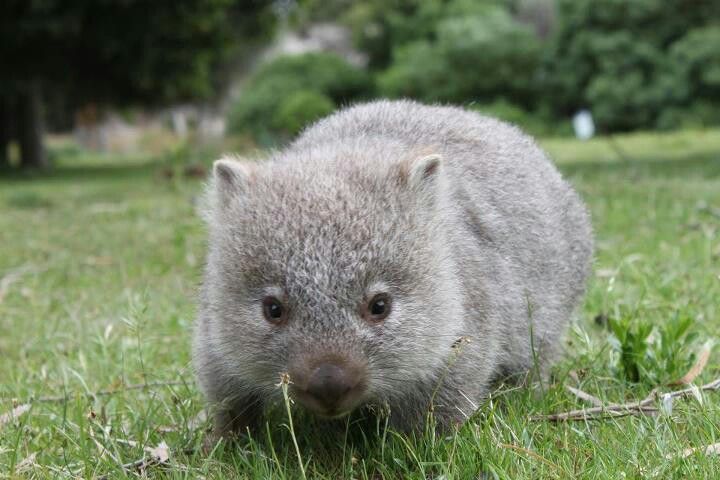
JavaScript Constructor Function
A basic tutorial on creating a JavaScript Constructor Function.
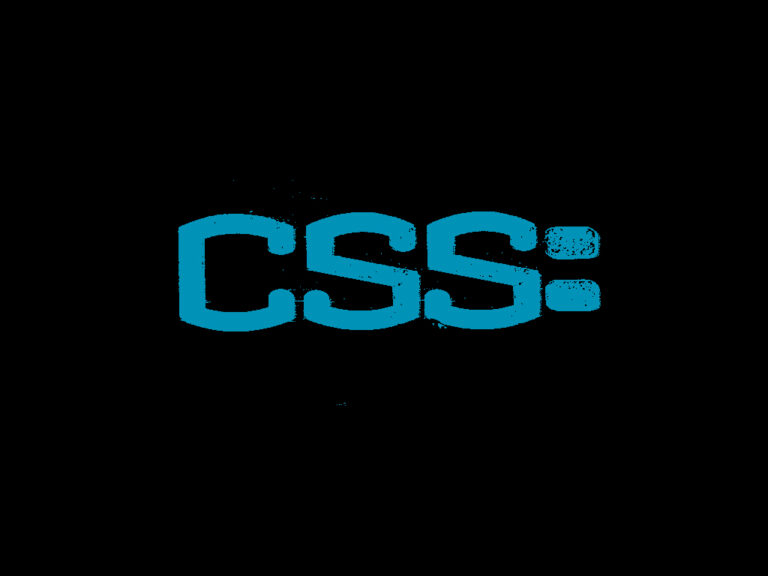
The Case For External CSS
SCENARIO 1: REPEAT, REPEAT AGAIN You start writing some html for a single web page.…
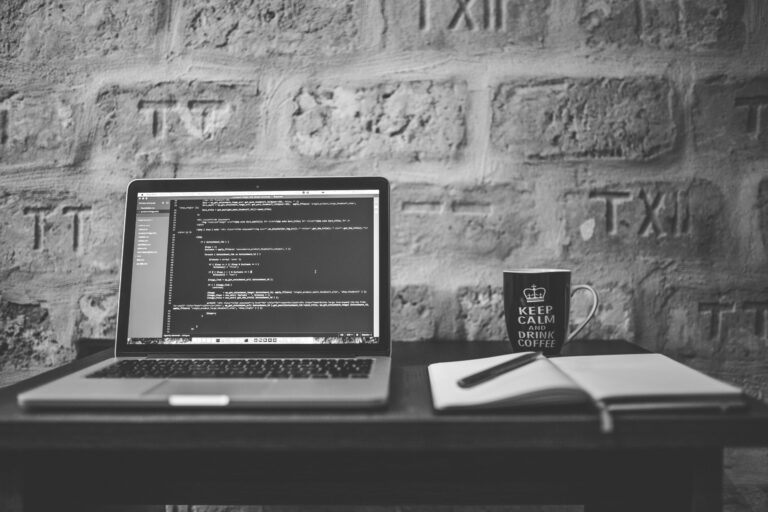
Web Design Basics
Much could be said about modern web design. Courses have been written and videos produced.…
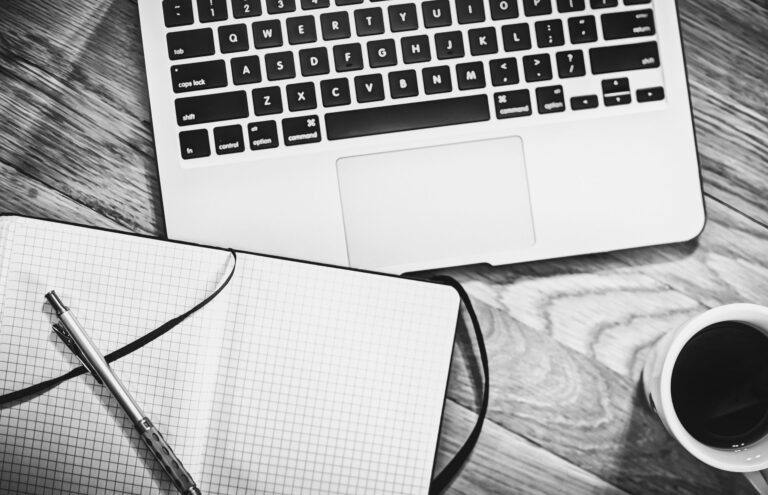
CSS Position
When building a webpage, understanding how to position elements correctly is key to creating a…
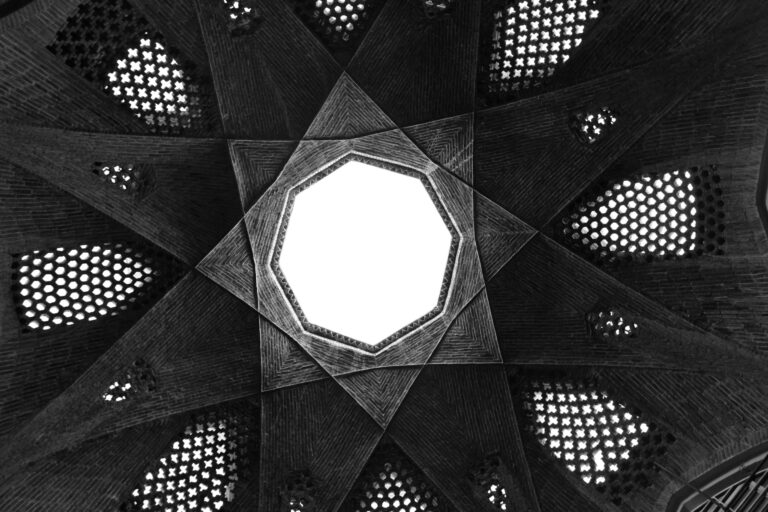
CSS Cursors
CSS enables you to customize the cursor’s appearance, enhancing user interaction by signaling specific actions.…
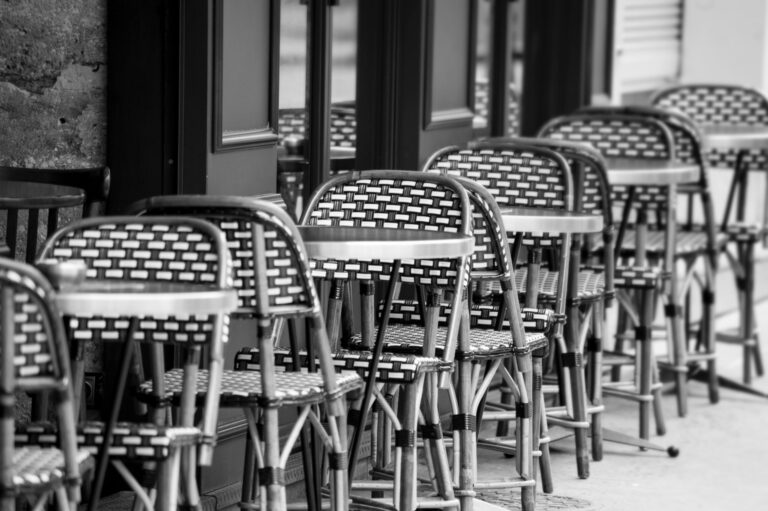
CSS Tables
Tables are a great way to display structured data, but by default, they can look…
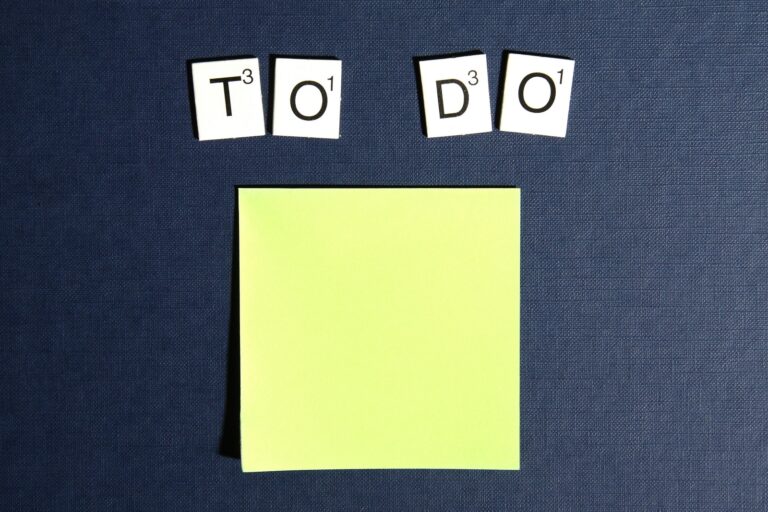
CSS Lists
Lists are a fundamental part of web design, helping to organize content in an easy-to-read…
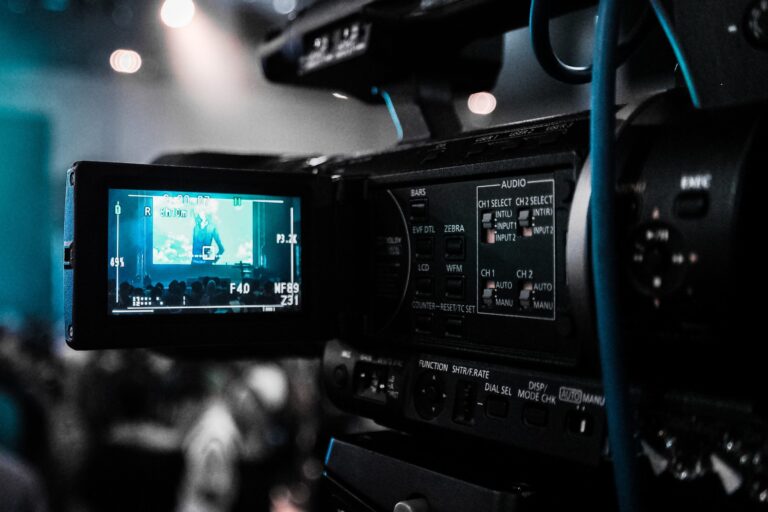
CSS Color
Intro to CSS Colors Colors are an important aspect of web design. They can help…
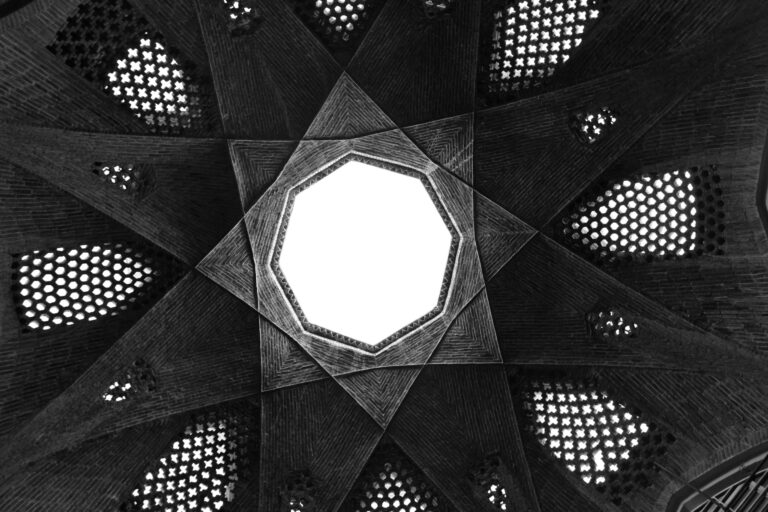
CSS Text
Introduction CSS provides powerful tools to control the appearance of text on a webpage. From…
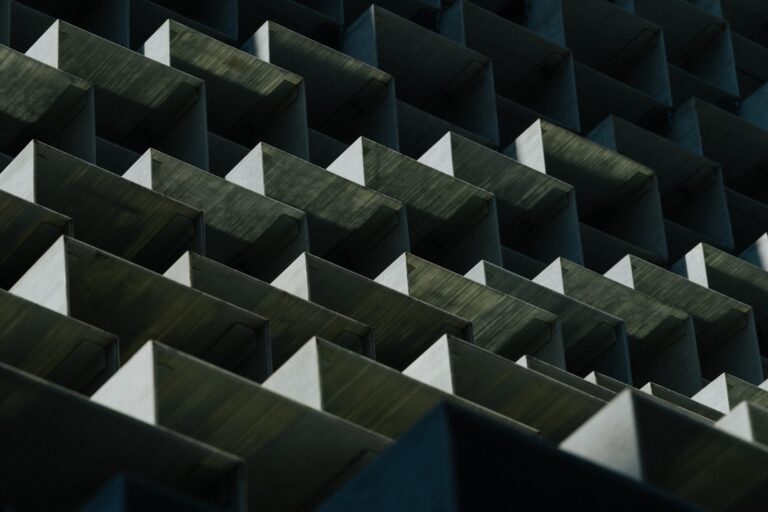
CSS Boxes
Introduction When working with CSS, every element on a webpage is treated as a box.…
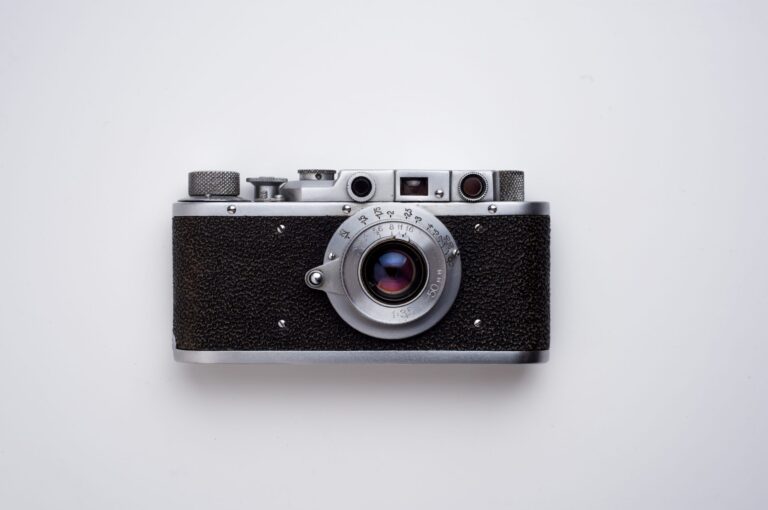
Intro to CSS
What is CSS? CSS stands for Cascading Style Sheet and is what we use to…
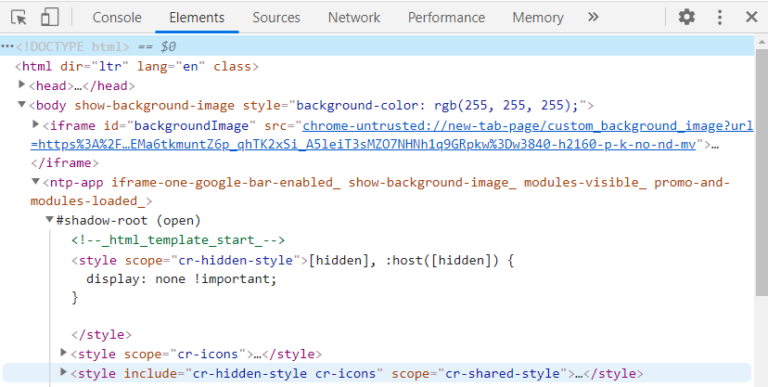
Inspecting Source Code in the Browser
All modern browsers have developer tools that allow you to inspect the source code. For…
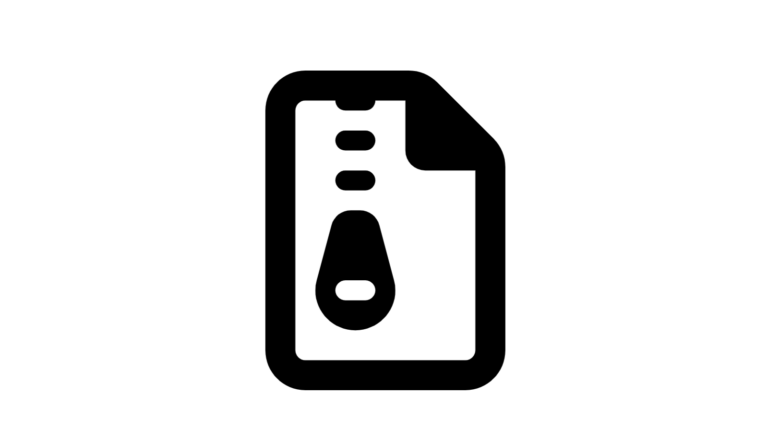
How to Zip a folder
Windows skip to Mac Right click on the folder you want to zipClick on “Send…